什么是区块链交易?
区块链交易指的是在区块链上进行的转移数字货币的过程。在区块链交易中,交易双方会创建一条交易记录,并用自己的私钥对交易进行数字签名。随后,这条交易记录会被广播到整个网络中的节点,并被记录在一个新的区块中。这个区块会被加入到区块链的末尾并被称为新的区块,交易流程也就完成了。
PHP如何实现区块链交易?
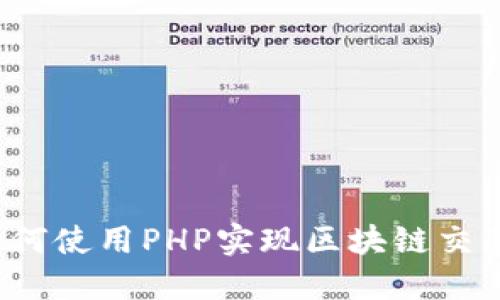
PHP是一种常用的后端编程语言,可以与区块链进行交互实现数字货币的交易。通常,需要使用PHP所提供的加密和哈希函数、JSON库等相关功能。具体实现过程如下:
1.生成钱包地址以及公钥和私钥。
2.连接至区块链的节点并创建货币交易。
3.用私钥对交易进行签名,并发送到网络节点进行广播。
4.等待交易被区块链网络确认。
5.根据可用余额进行存储,更新余额记录。
如何进行PHP和区块链的交互?
要想实现PHP和区块链的交互,需要使用相关的API和库文件。可以使用比特币核心(Bitcoin Core)的RPC接口来与区块链进行交互。这里介绍一个常用的PHP框架Bitcoin PHP,可以使用composer进行安装,在composer.json中添加以下依赖即可:
``` { "require": { "bitwasp/bitcoin": "dev-master", "tuupola/base58": "1.*" } } ```如何创建交易?
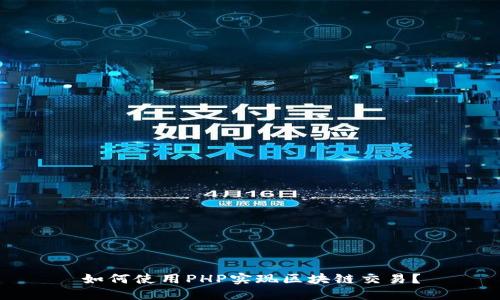
在Bitcoin PHP中,可以使用TransactionFactory类来创建交易。交易分为发送方和接收方,需要指定from(发送方)、to(接收方)、amount(金额)和fee(交易费用)。使用TransactionFactory类的create方法可以创建一个交易,如下所示:
``` use BitWasp\Bitcoin\Address\PayToPubKeyHashAddress; use BitWasp\Bitcoin\Script\ScriptFactory; use BitWasp\Bitcoin\Transaction\TransactionFactory; $useTestnet = false; $fromAddress = new PayToPubKeyHashAddress('1AtxvqZVwYwFETG2LjWsVLg2zXhcDfutbT'); $toAddress = new PayToPubKeyHashAddress('1Co6tTaJ8syhoFXanMZEkjJXeZPMfysLKY'); $amount = 10000; $fee = 1000; $transaction = TransactionFactory::create() ->input('test', 0) ->payToAddress($amount, $toAddress) ->payToAddress($fromAddress->getAddress(), $fee) ->get(); ```如何签名交易?
要对交易进行签名,需要使用私钥和交易的事务数据。在Bitcoin PHP中,可以使用Key类来进行签名。具体代码如下:
``` use BitWasp\Bitcoin\Key\PrivateKeyFactory; $privateWif = 'L4o2d4sLHDAnCqQh1ga9JvSjzxx6u5VpkWva44Fs3angQJQjUrLj'; $private = PrivateKeyFactory::fromWif($privateWif); $transaction->input(0, $scriptPubKey) ->sign($private); ```如何广播交易?
通过使用Bitcoin Core的RPC接口,可以将交易广播到区块链网络。需要使用RPC调用来进行这一操作,如下所示:
``` use BitWasp\Bitcoin\Client\BitcoinClient; use BitWasp\Bitcoin\Config\Configuration; $configuration = new Configuration(); $configuration->setHost('localhost'); $configuration->setPort(8332); $configuration->setUser('username'); $configuration->setPassword('password'); $client = new BitcoinClient($configuration); $client->sendrawtransaction($transaction->getHex()); ```如何同步交易记录?
在区块链交易中,需要同步最新的交易记录,以便更新钱包余额和交易历史记录。使用Bitcoin PHP的Block Explorer(bech32)类可以获取区块头和交易信息。如下所示,$hash为交易的哈希值:
``` use BitWasp\Bitcoin\Client\Bitcoind; use BitWasp\Bitcoin\Math\Math; use BitWasp\Bitcoin\Transaction\TransactionBuilderFactory; use BitWasp\Bitcoin\Block\Locator; use BitWasp\Bitcoin\Block\Block; use BitWasp\Bitcoin\Chain\Hashes; $bitcoind = new Bitcoind('localhost', 'user', 'password'); $math = new Math(); $locator = new Locator($bitcoind->getBlockchainInfo(), $math); $blockHashes = $locator->getHashes(); $blockHashes[] = $hash; $lastHash = null; $blocks = []; foreach (array_reverse($blockHashes) as $hash) { $block = Block::fromHex($bitcoind->getBlock($hash)); $blocks[$hash] = [ 'block' => $block, 'hash' => $hash, ]; if ($lastHash !== null) { $blocks[$hash]['next'] = $lastHash; } $lastHash = $hash; } $hashes = new Hashes($math); $locator = new Locator($bitcoind->getBlockchainInfo(), $math); $locator_testnet = new Locator($bitcoind->getBlockchainInfo(), $math, true); $blockIndexer = $bitcoind->getBlockIndexer(); foreach ($blocks as $id =>